Object-oriented programming (OOP) is a programming technique that combines data abstraction and inheritance. The central feature is the object, which in practical terms is a development of a data structure. The latter is a composite data type, consisting of a collection of appropriate variables. The object comprises a data structure definition and its defined procedures in a single structure.
Objects are instances of a class, each instance having its own private instance variables. The class definition defines the properties of the objects in a class. A particularly important feature is inheritance, which allows new classes to be defined in terms of existing classes, inheriting some or all of the properties of an existing class. This facilitates sharing of code, since users can inherit objects from system collections of code.
The procedures of an object (often called methods) are activated by messages sent to the object by another object. Thus in an object-oriented programming system the basic control structure is message passing. The programmer identifies the real-world objects of the problem and the processing requirements of those objects, encapsulating these in class definitions, and the communications between objects. The program is then essentially a simulation of the real world in which objects pass messages to other objects to initiate actions. The interior structure of the object is entirely hidden from any other object (a property called encapsulation).
Objects add several capabilities to the data structure. The most important are briefly described below.
Procedures
Objects can contain procedures (methods) as well as variables (also called properties). For example, a data type representing an automobile might be defined in C as follows:
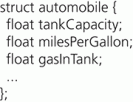
Variables of type ‘automobile’ would then be instantiated, initialized by assigning appropriate values to their component subvariables, and manipulated by appropriate procedures. In OOP, the automobile structure could be reformulated in C++ to include a procedure:
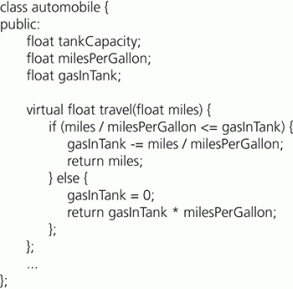
Assuming a variable of type ‘automobile’ called ‘gasGuzzler,’ the method ‘travel’ could be called thus:

(The significance of the keyword ‘virtual’ is explained below.)
Inheritance
The fact that objects can be defined in terms of other objects is an important feature of OOP. A ‘child’ object type (or subclass) inherits all of its parent’s methods and properties (which may have been inherited in turn from its parent) as well as defining its own. This facility allows complex hierarchies of related objects to be created. A child object type may override a method or property of its parent by defining a new method or property of the same name:
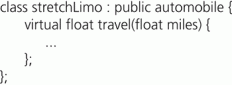
If the ‘travel’ method of a variable of type ‘stretchLimo’ is called, it is stretchLimo’s own version that is invoked rather the version inherited from class ‘automobile.’
Child object types may be treated as if they are one of their ancestor types. The following is a legal assignment using C++ pointers:

No data is lost, but only those properties and methods defined for class ‘automobile’ will be available to variable myLimo.
Polymorphism
if a method has been overridden, it is usually the overriding method defined in a child class that is invoked. For example,

will invoke the ‘travel’ method defined in class stretchLimo because ‘myLimo’ is in fact a stretchLimo object. (Note that in C++ methods must be designated ‘virtual’ to achieve this behaviour; in such languages as Java it is automatic.)
This capability, called polymorphism, gives object-oriented programming its great power. The class ‘automobile’ could have numerous subclasses defined, each extending it in ways appropriate to a particular brand of automobile; and each might have its own version of the ‘travel’ method, tuned to suit its own characteristics. Yet, when an object of any subclass is assigned to pointer variable of class ‘automobile’ and the ‘travel’ method invoked, it is the variant defined for the appropriate subclass that is invoked. Each object thus carries the knowledge of how to manipulate itself, and the code that uses such objects need not be aware of exactly which type of object it is manipulating. This enhances encapsulation, reducing the complexity and increasing the robustness of almost any nontrivial programming project.
An important application of polymorphism is in interfaces. These may be defined as abstract classes: that is, classes that define properties and methods but which do not provide any implementations for the methods. An implementation of an interface is a subclass that overrides all the interface’s methods with versions that provide appropriate functionality.
Languages
The first complete realization of an object-oriented programming system was Smalltalk. A more recent example is Ruby. In addition a large number of modern programming languages contain features of object-oriented systems; examples are C++, C#, Java, and Visual Basic.